아래 내용을 참고하여 원을 나타내는 Circle 클래스를 만들고 테스트해 보자.
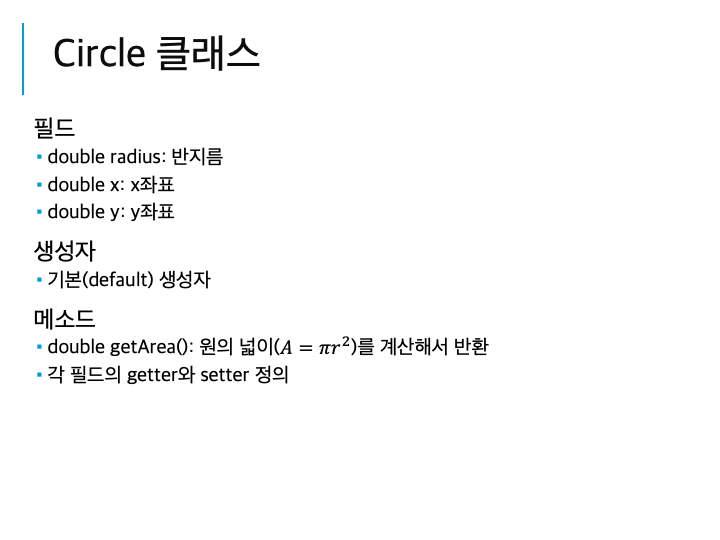
package practice;
public class Circle {
// 필드
private double radius;
private double x;
private double y;
// 생성자
public Circle() {
}
Circle(double radius, double x, double y) {
this.radius = radius;
this.x = x;
this.y = y;
}
// 메소드
public double getArea() {
return radius * radius * Math.PI;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
if (radius > 0) {
this.radius = radius;
}
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
package practice;
public class CircleTest {
public static void main(String[] args) {
Circle circle1 = new Circle();
circle1.setRadius(3.6);
circle1.setX(0.0);
circle1.setY(1.5);
System.out.println("반지름: "+ circle1.getRadius());
System.out.println("중심좌표: "+"("+ circle1.getX() + ","+ circle1.getY()+")");
System.out.printf("넓이: %.2f\n", circle1.getArea());
Circle circle2 = new Circle();
circle2.setRadius(-5.0);
circle2.setX(1.0);
circle2.setY(2.2);
System.out.println("반지름: "+ circle2.getRadius());
System.out.println("중심좌표: "+"("+ circle2.getX() + ","+ circle2.getY()+")");
System.out.printf("넓이: %.2f\n", circle2.getArea());
}
}
'Java > 과제' 카테고리의 다른 글
chapter06) 클래스 example - Plane (0) | 2022.08.24 |
---|---|
chapter06) 클래스 example - Time (0) | 2022.08.24 |
chapter05) 참조타입 example - 주사위를 던져서 각 면이 나오는 횟수를 출력하는 프로그램 (0) | 2022.08.17 |
chapter05) 참조타입 example - 인공지능 컴퓨터와 게이머(사람)가 가위바위보 게임을 할 수 있는 프로그램 (0) | 2022.08.17 |
chapter05) 참조타입 example - 로또번호를 생성하는 프로그램 (0) | 2022.08.17 |