아래 내용 클래스 다이어그램과 내용을 참고하여 Car 클래스를 NewCar 클래스로 새롭게 만들고 테스트 해 보자.
테스트 코드는 앞의 Car 클래스에서 테스트했던 내용을 그대로 사용하여 결과가 동일하게 나오는지 확인한다.
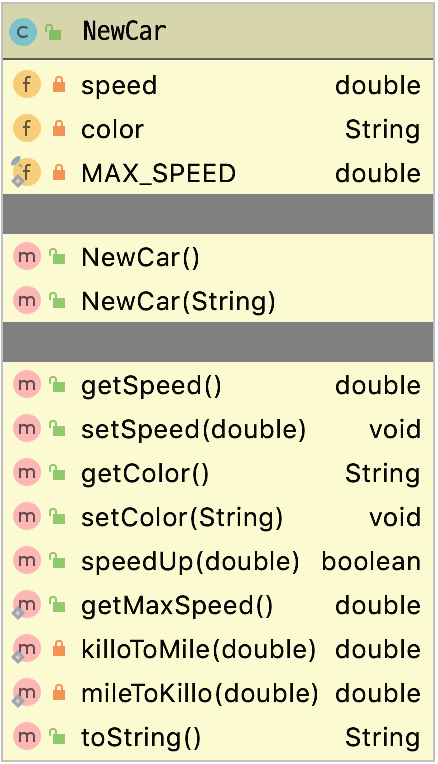
package practice;
public class NewCar {
// 필드
private double speed;
private String color;
private static final double MAX_SPEED = KilloToMile(200.0);
// 생성자
public NewCar() {
}
NewCar(String color) {
this.color = color;
}
// 메소드
public double getSpeed() {
return MileToKillo(speed);
}
public void setSpeed(double speed) {
this.speed = KilloToMile(speed);
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public boolean speedUp(double speed) {
if (this.speed + KilloToMile(speed) < 0 || this.speed + KilloToMile(speed) > MAX_SPEED) {
return false;
} else {
this.speed += KilloToMile(speed);
return true;
}
}
public static double getMaxSpeed() {
return MileToKillo(MAX_SPEED);
}
static double KilloToMile(double distance) {
return distance / 1.6;
}
package practice;
public class NewCarTest {
public static void main(String[] args) {
NewCar myCar = new NewCar("red");
System.out.println("myCar의 색: " + myCar.getColor());
System.out.println("차의 최대 속력: " + Car.getMaxSpeed() + "㎞/h");
System.out.print("첫 번째 speedUp(100.0㎞/h): ");
if (myCar.speedUp(100.0)) {
System.out.print("속도 변경 가능,");
} else {
System.out.print("속도 변경 불가능,");
}
System.out.println(" 현재 차의 속력: " + myCar.getSpeed() + "㎞/h");
System.out.print("두 번째 speedUp(90.0㎞/h): ");
if (myCar.speedUp(90.0)) {
System.out.print("속도 변경 가능,");
} else {
System.out.print("속도 변경 불가능,");
}
System.out.print(" 현재 차의 속력: " + myCar.getSpeed() + "㎞/h");
Car yourCar = new Car("blue");
System.out.println();
System.out.println("yourCar의 색: " + yourCar.getColor());
System.out.println("차의 최대 속력: " + Car.getMaxSpeed() + "㎞/h");
System.out.print("첫 번째 speedUp(-100.0㎞/h): ");
if (yourCar.speedUp(-100.0)) {
System.out.print("속도 변경 가능, ");
} else {
System.out.print("속도 변경 불가능, ");
}
System.out.println(" 현재 차의 속력: " + yourCar.getSpeed() + "㎞/h");
System.out.print("두 번째 speedUp(210.0㎞/h): ");
if (yourCar.speedUp(210.0)) {
System.out.print("속도 변경 가능, ");
} else {
System.out.print("속도 변경 불가능, ");
}
System.out.print(" 현재 차의 속력: " + yourCar.getSpeed() + "㎞/h");
}
}
'Java > 과제' 카테고리의 다른 글
chapter 07) 상속example - 도형 관련 클래스 만들기 (0) | 2022.08.26 |
---|---|
chapter 07) 상속example - Student 클래스 만들기 (0) | 2022.08.26 |
chapter06) 클래스 example - Car (0) | 2022.08.24 |
chapter06) 클래스 example - Plane (0) | 2022.08.24 |
chapter06) 클래스 example - Time (0) | 2022.08.24 |